Continuing on from Part 1 of this tutorial, let's play a little with XML data using the HEntry techniques discussed.
Here is some example XML that we'll play with:
<teams>
<team name="Stark">
<sigil>Direwolf</sigil>
<member position="pitcher">Robb</member>
<member position="crowsnest">Bran</member>
</team>
<team name="Lannister">
<sigil>Lion</sigil>
<member position="pitcher">Jaime</member>
<member position="bat boy">Lancel</member>
<member position="solar">Kevan</member>
</team>
</teams>
To handle the XML in TDI you could read in a file. A handier approach for play/test is to set up an AL with the FormEntry Connector and paste the encoded string into the Raw Data Text parameter:
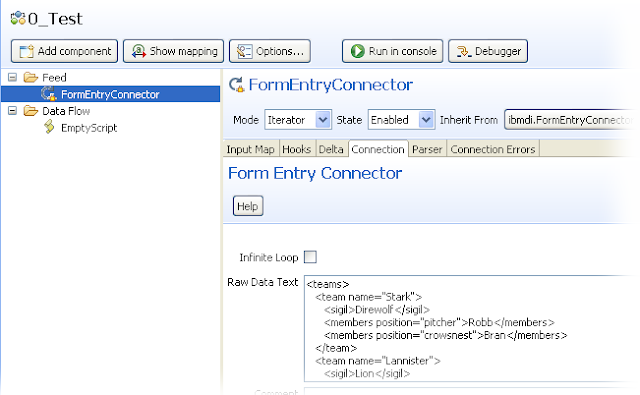
FormEntry works like a File Connector, but instead of reading from a file, it uses the data entered into the Raw Data Text parameter.
Add the wildcard map (* map all attributes) to the Input Map and then choose the XML Parser, clearing out the Entry Tag parameter. This tells the parser to return a single Hentry.
From the topmost screenshot you can see that I also dropped an Empty Script in the Data Flow section of my AL. Right click on this empty script and select Run and break here. This launches the AL in the Debugger and runs to this point.
The Watch List (off to the right) shows you that the data is parsed and there are attributes available in the Work Entry. We will ignore this, since it's only part of the truth.
At this point we can start playing with the Hentry. Lets start by accessing the root node of the hierarchical data: teams. Do this by typing work.teams in the Javascript command line and then pressing Enter. This executes the snippet of script and displays the results in the log output view.
The Debugger first displays the snippet that was executed, followed by >> and then the result. The output above shows this is a TDI Attribute. Attributes display themselves first with their name in quotes followed by a colon (:) and then the value. In the above screenshot the square brackets shown for the value tells us this is a hierarchical Attribute. To get at the children we can use the getChildNodes() method:
children = work.teams.getChildNodes()
The Debugger tells us that we get a NodeList back (forget the Immutable bit). A NodeList is a standard w3c object that holds a list of nodes: http://docs.oracle.com/javase/6/docs/api/org/w3c/dom/NodeList.html
A NodeList has only two methods: getLength() and item(). The first one tells you how many nodes are in the list, and the second one is for accessing a node. For example:
for (i = 0; i < children.getLength(); i++) task.logmsg(children.item(i))
This time the output is too long for the log view. So you can press the Page button to view the entire log output in a separate editor.
Note also that instead of using the .item() method, you can also just use square brackets:
task.logmsg(children[i]);
And furthermore, you can use the for-in construct as well to enumerate child nodes:
for (child in children) task.logmsg(child.toString());